mirror of
https://github.com/comfyanonymous/ComfyUI.git
synced 2025-01-11 18:35:17 +00:00
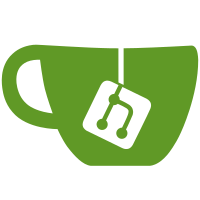
* menu * wip * wip * wip * wip * wip * workflow saving/loading * Support inserting workflows Move buttosn to top of lists * fix session storage implement renaming * temp * refactor, better workflow instance management * wip * progress on progress * added send to workflow various fixes * Support multiple image loaders * Support dynamic size breakpoints based on content * various fixes add close unsaved warning * Add filtering tree * prevent renaming unsaved * fix zindex on hover * fix top offset * use filename as workflow name * resize on setting change * hide element until it is drawn * remove glow * Fix export name * Fix test, revert accidental changes to groupNode * Fix colors on all themes * show hover items on smaller screen (mobile) * remove debugging code * dialog fix * Dont reorder open workflows Allow elements around canvas * Toggle body display on setting change * Fix menu disappearing on chrome * Increase delay when typing, remove margin on Safari, fix dialog location * Fix overflow issue on iOS * Add reset view button Prevent view changes causing history entries * Bottom menu wip * Various fixes * Fix merge * Fix breaking old menu position * Fix merge adding restore view to loadGraphData
44 lines
1.3 KiB
JavaScript
44 lines
1.3 KiB
JavaScript
// @ts-check
|
|
|
|
import { $el } from "../../ui.js";
|
|
import { ComfyButton } from "./button.js";
|
|
import { prop } from "../../utils.js";
|
|
import { ComfyPopup } from "./popup.js";
|
|
|
|
export class ComfySplitButton {
|
|
/**
|
|
* @param {{
|
|
* primary: ComfyButton,
|
|
* mode?: "hover" | "click",
|
|
* horizontal?: "left" | "right",
|
|
* position?: "relative" | "absolute"
|
|
* }} param0
|
|
* @param {Array<ComfyButton> | Array<HTMLElement>} items
|
|
*/
|
|
constructor({ primary, mode, horizontal = "left", position = "relative" }, ...items) {
|
|
this.arrow = new ComfyButton({
|
|
icon: "chevron-down",
|
|
});
|
|
this.element = $el("div.comfyui-split-button" + (mode === "hover" ? ".hover" : ""), [
|
|
$el("div.comfyui-split-primary", primary.element),
|
|
$el("div.comfyui-split-arrow", this.arrow.element),
|
|
]);
|
|
this.popup = new ComfyPopup({
|
|
target: this.element,
|
|
container: position === "relative" ? this.element : document.body,
|
|
classList: "comfyui-split-button-popup" + (mode === "hover" ? " hover" : ""),
|
|
closeOnEscape: mode === "click",
|
|
position,
|
|
horizontal,
|
|
});
|
|
|
|
this.arrow.withPopup(this.popup, mode);
|
|
|
|
this.items = prop(this, "items", items, () => this.update());
|
|
}
|
|
|
|
update() {
|
|
this.popup.element.replaceChildren(...this.items.map((b) => b.element ?? b));
|
|
}
|
|
}
|